by TechiBee
on August 12, 2011
Are you in need of a script which uninstall patches from both local and remote computers? You landed at right place. Today I came across a question in one of the forum asking for a way to uninstall patches/security updates/hotfixes from llocal or remote computers. While this is an easy thing to perform on local computers, it is little tricky when it comes to remote computers. I thought about it some time and finally came up with below code which works for both local and remote computers. All it does is, constructs a uninstall command for the given patch and executes that using WMI process class.
This script comes handy for you when you want to uninstall patches from Windows 2008 Core server which don’t have a GUI and can’t perform uninstallations the way you do in regular windows 2008 OS.
Here is the code:
function Uninstall-Hotfix {
[cmdletbinding()]
param(
$computername = $env:computername,
[string] $HotfixID
)
$hotfixes = Get-WmiObject -ComputerName $computername -Class Win32_QuickFixEngineering | select hotfixid
if($hotfixes -match $hotfixID) {
$hotfixID = $HotfixID.Replace("KB","")
Write-host "Found the hotfix KB" + $HotfixID
Write-Host "Uninstalling the hotfix"
$UninstallString = "cmd.exe /c wusa.exe /uninstall /KB:$hotfixID /quiet /norestart"
([WMICLASS]"\\$computername\ROOT\CIMV2:win32_process").Create($UninstallString) | out-null
while (@(Get-Process wusa -computername $computername -ErrorAction SilentlyContinue).Count -ne 0) {
Start-Sleep 3
Write-Host "Waiting for update removal to finish ..."
}
write-host "Completed the uninstallation of $hotfixID"
}
else {
write-host "Given hotfix($hotfixID) not found"
return
}
}
Usage:
Uninstall-HotFix -ComputerName PC1 -HotfixID KB123456
{ }
by TechiBee
on August 12, 2011
Querying the user lists who interactively logged on to a computer. I want to do this from very long time using powershell so that I can use it in my other powershell scripts. Earlier I tried to do it via WMI but I was not very successful. After struggling for some time, I finally made a code which works for most windows platforms from which you can query interactively logged on users information.
This script is a wrapper which depends on most famous psloggedon utility. It parses the output of the psloggedon util and prepares a object with domain and user information.
function Get-LoggedOnUsers {
[cmdletbinding()]
param(
[parameter(valuefrompipelinebypropertyname=$true)]
[string]$ComputerName = $env:computername
)
begin {}
process {
[object[]]$sessions = Invoke-Expression "PsLoggedon.exe -x -l \$ComputerName 2> null" |
Where-Object {$_ -match '^s{2,}((?w+)\(?S+))'} |
Select-Object @{
Name='Computer'
Expression={$ComputerName}
},
@{
Name='Domain'
Expression={$matches.Domain}
},
@{
Name='User'
Expression={$Matches.User}
}
IF ($Sessions.count -ge 1)
{
return $sessions
}
Else
{
'No user logins found'
}
}
end {}
}
I found this code originally at Scripting Guys blog. I had to make few modifications to make it parse the output properly and exclude the psloggedon util logo information.
{ }
by TechiBee
on August 10, 2011
PowerShell has a native module called “ActiveDirectory” using which we can query active directory. The advantage of using this is, you no need to depend on external installers like Quest PowerShell tools to query active directory. The only pre-requisites for using the cmdlets in ActiveDirectory module is you should run these from either vista or windows 7 computer and you environment should have atleast one Windows 2008 R2 DC(or should have ADWS installed on windows 2003 DCs).
So, here is the code to query active directory group for its members.
Import-Module ActiveDirectory
Get-ADGroupMember -Identity Group1 | select Name, ObjectClass
In the above code I am first importing ActiveDirectory module and the using Get-ADGroupMember cmdlet to query the group named Group1
If the Group1 has some more groups inside it, you can query the group recursively using the -recurse parameter.
ActiveDirectory some other useful cmdlets which you can use to manage group membership of Groups. For example, Add-ADGroupMember cmdlet helps us in adding a object to a group in AD. Similarly using Remove-ADGroupMeber we can remove objects from a group.
{ }
by TechiBee
on August 9, 2011
After you install Windows 2008 R2 Core Server from media, you notice that powershell is installed by default. PowerShell is essential in Core environment to administer core operating system. So, it is recommended to install powershell after you install Core operating system to take full advantage of this powerful programming language in configuring the several OS parameters. Today, I installed a core server and thought of writing a articles on how to install powershell in Core.
Procedure to install powershell in Windows 2008 R2 Server Core:
- Logon to the Server with administrator account and start server configuration wizard by typing “SCONFIG” in command prompt.

- Select option “4” in Server Configuration wizard to enter into “Configure Remote Management”

- Select option “2” to install powershell (Enable Windows PowerShell)

- Reboot the server if prompted

This completes the installation. Now you can start powershell by starting the process “powershell.exe” either from command prompt or from task manager.
Hope this helps…
{ }
by TechiBee
on August 9, 2011
In this post I am going to detail about querying various file attributes using powershell and some more operations related to that. Get-Item cmdlet helps you to fetch this information. Along with creationtime, it provides some details about the file you are querying. Let us go and see what they are…
Get file creation time:
(Get-item C:localadmincred.xml).creationtime
Get file modification time:
(Get-item C:localadmincred.xml).lastwritetime
Get file size:
"{0:N2}" -f ((Get-item C:localadmincred.xml).length/1mb) + " MB"
Get file extension:
Get-item C:localadmincred.xml | select extension
Get the directory name where file file is located:
dmincred.xml | select directory
Get file Security permissions:
(Get-item C:localdemo.xml).getaccesscontrol.invoke() | select owner -ExpandProperty access
Hope this helps…
{ }
by TechiBee
on August 9, 2011
This little powershell function allows you to get the size of given folder. It has two parameters, -Name which takes file path as argument and -recurse which gets you size of the folders including the recursive items. For this powershell function -name is mandatory parameter and can take values from pipeline by property name.
function Get-FolderSize {
[CmdletBinding()]
param(
[parameter(valuefrompipelinebypropertyname=$true,mandatory=$true)]
$Name,
[switch]
$recurse
)
begin {}
process {
if($recurse) {
$colItems = (Get-ChildItem $name -recurse | Measure-Object -property length -sum)
"Size of $name is -- " + "{0:N2}" -f ($colItems.sum / 1GB) + " GB"
}
else {
$colItems = (Get-ChildItem $name | Measure-Object -property length -sum)
"Size of $name is -- " + "{0:N2}" -f ($colItems.sum / 1GB) + " GB"
}
}
end {}
}
Output:
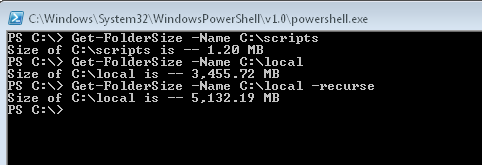
{ }
by TechiBee
on August 7, 2011
$file1version = [version](Get-Command .File1.dll).FileVersionInfo.FileVersion
$file2version = [version](Get-Command .File2.dll).FileVersionInfo.FileVersion
if($file1version -eq $file2version) {
write-host "File version numbers are equal"
} else {
write-host "File version numbers are not equal"
}
Above simple code compares file versions of two files. The comparison here is very straight forward. In the above code it first queries the file versions of two files, File1 and File2 and compares both of them to see if they are equal or not.
function Get-FileVersionInfo
{
param(
[Parameter(Mandatory=$true)]
[string]$FileName)
if(!(test-path $filename)) {
write-host "File not found"
return $null
}
return [System.Diagnostics.FileVersionInfo]::GetVersionInfo($FileName)
}
Above code is little bit enhanced which checks for file existence and returns the version numbers in object format which can be further used for other version control related operations.
{ }
by TechiBee
on July 13, 2011
Assigning a static IP address is one common task that system administrators do after building a server. In today’s post I am providing a powershell function(Set-StaticIP) which takes IP address, subnet mask, default gateway and DNS servers as parameters and assigns it to the computer where you are executing the function.
function Set-StaticIP {
<# .Synopsis Assings a static IP address to local computer. .Description Assings a static IP address to local computer. .Parameter IPAddress IP Address that you want to assign to the local computer. .Parameter Subnetmask Subnet Mask that you want to assign to the computer. This is optional. If you don't specify anything, it defaults to "255.255.255.0". .Parameter DefaultGateway Default Gateway IP Address that you want to assign to the local computer. .Parameter DNSServers DNS servers list that you want to configure in network connection properties. .Example Set-StaticIP -IPAddress 10.10.10.123 -DefaultGateay 10.10.10.100 -DNSServers 10.10.10.120,10.10.10.121 .Notes NAME: Set-StaticIP AUTHOR: Sitaram Pamarthi Website: www.techibee.com #>
[CmdletBinding()]
param (
[alias('IP')]
[Parameter(mandatory=$true)]
[string]$IPAddress,
[alias('Sb')]
[Parameter(mandatory=$false)]
[string]$Subnetmask = "255.255.255.0",
[alias('Gateway')]
[Parameter(mandatory=$true)]
[string]$DefaultGateway,
[alias('DNS')]
[parameter(mandatory=$false)]
[array]$DNSservers
)
Get-WmiObject -Class Win32_NetworkAdapterConfiguration -Filter "IPenabled=TRUE" | % {
Write-host "Trying to set IP Address on $($_.Description)"
$_.EnableStatic($IPAddress,$Subnetmask) | out-null
$_.SetGateways($DefaultGateway) | out-null
$_.SetDNSServerSearchOrder($DNSServers) | out-null
}
if($?) {
write-host "IP Address set successfully"
} else {
write-host "Static IP assignment failed"
}
}
Above simple function assigns the IP address and displays the output of execution.
Feel free to post here if you have any questions about the script.
Happy learning.
{ }
by TechiBee
on July 12, 2011
System Center Virtual Machine Manager(SCVMM) is helps in managing physical and virtual IT infrastructure from central place. You can read about SCVMM at http://www.microsoft.com/systemcenter/en/us/virtual-machine-manager.aspx
SCVMM has powershell interface also which enables IT administrators to automate tasks. There are variety list of cmdlets related to SCVMM are available. You can download reference guide from here.
{ }
by TechiBee
on July 11, 2011
I inspired from my previous post, and decide to do some network interface related operations from command line as they helps me when managing Windows 2008 Core Operating system. Another command that I am going to provide now is to disable network connection from command line.
netsh interface set interface name=”Local Area Connection 1″ admin=DISABLED
In about command, “Local Area Connection 1” is the name of the connection that you want to disable. You can change the value of “Admin” to “Enable” to enable back the network connection. Similarly, if you want to rename the network connection, you can use newname parameter. Below is the command.
netsh interface set interface name=”Local Area Connection 1″ newname=”My NIC1″
Above command renames “Local Area Connection 1” network to “My NIC1”.
Hope this helps…
{ }