by TechiBee
on August 17, 2010
Today I got a requirement to restart a service on remote computer. I thought of doing it with powershell using restart-service powershell cmdlet but it disappointed me. Though Get-Service cmdlet is offering -computername parameter, stop-service, start-service, and restart-service cmdlets are not offering this feature. That means you can query service status on remote PC but cannot start/stop with built-in facilities/cmdlets.
However, there is a way to do it using WMI in combination with powershell.
(Get-WMIObject -computername remotepc -class win32_service "Name='alerter'").stopservice()
(Get-WMIObject -computername remotepc -class win32_service "Name='alerter'").startservice()
There is no direct method available to restart a service directly, but you can achieve similar functionality with stop followed by start method which makes sense.
You like to know what more we can do here? Just issue below command.
(Get-WMIObject -computername remotepc -class win32_service "Name='alerter'") | gm
{ }
by TechiBee
on August 12, 2010
Updated on 2/13/2012 with a more robust function to get OS architecture and details.
After introduction of windows 7 and 2008, use of 64-bit operating systems have increased. Today I came across a requirement to generate list of servers which are running with 32-bit operating system and 64-bit operating system. I look at OperatingSystem attribute value of active directory objects, but that doesn’t have sufficient information. I did bit more research and came-up with below powershell script which works for windows XP, 2003, 7, 2008.
Code:
function Get-OSArchitecture {
[cmdletbinding()]
param(
[parameter(ValueFromPipeline=$true,ValueFromPipelineByPropertyName=$true)]
[string[]]$ComputerName = $env:computername
)
begin {}
process {
foreach ($Computer in $ComputerName) {
if(Test-Connection -ComputerName $Computer -Count 1 -ea 0) {
Write-Verbose "$Computer is online"
$OS = (Get-WmiObject -computername $computer -class Win32_OperatingSystem ).Caption
if ((Get-WmiObject -Class Win32_OperatingSystem -ComputerName $Computer -ea 0).OSArchitecture -eq '64-bit') {
$architecture = "64-Bit"
} else {
$architecture = "32-Bit"
}
$OutputObj = New-Object -Type PSObject
$OutputObj | Add-Member -MemberType NoteProperty -Name ComputerName -Value $Computer.ToUpper()
$OutputObj | Add-Member -MemberType NoteProperty -Name Architecture -Value $architecture
$OutputObj | Add-Member -MemberType NoteProperty -Name OperatingSystem -Value $OS
$OutputObj
}
}
}
end {}
}
Usage:
Get-OSArchitecture -ComputerName PC1, PC2, PC3 | ft -auto
Hope this helps..
{ }
by TechiBee
on August 11, 2010
Description:
This powershell function helps you pinning the specified program to Task bar in windows 7/Windows Vista/Windows 2008 computers.
Usage:
[PS]:>Pinto-Taskbar -application “c:windowssystem32calc.exe”
The above statement Pins the calculator program to Task bar.
Code:
function pinto-taskbar {
param(
[parameter(Mandatory = $true)]
[string]$application
)
$appfolderpath = $application.SubString(0,$application.Length-($application.Split(“”)[$application.Split(“”).Count-1].Length))
$objshell = New-Object -ComObject “Shell.Application”
$objfolder = $objshell.Namespace($appfolderpath)
$appname = $objfolder.ParseName($application.SubString($application.Length-($application.Split(“”)[$application.Split(“”).Count-1].Length)))
$verbs = $appname.verbs()
foreach ($verb in $verbs) {
if($verb.name.replace(“&”,””) -match “Pin to Taskbar”) {
$verb.DoIt()
}
}
}
{ }
by TechiBee
on August 9, 2010
By saying “Graceful” close, I mean allowing the users to save any un saved data before exiting the application. It is equivalent to clicking on “CLOSE” button at the right top corner. The advantage with this method when compared “stop-process” is, it allows the user to save data and hence there is no data lose.
This option came handy to me several time.
Code:
Get-Process notepad | % { $_.CloseMainWindow() }
The above code will get list of notepad processes running in your PC and prompts you to save data for the processes which have unsaved content. The notepad processes which don’t have anything to save will get closed normally.
Hope this helps you…
{ }
by TechiBee
on August 7, 2010
Microsoft Office 2007 installation in windows 7 won’t create any Outlook Icon on User desktop. This is expected behavior with default installation. If you want to get this icon, then import the below registry information to your system and you should be able to see it immediately.
Windows Registry Editor Version 5.00
[HKEY_LOCAL_MACHINESOFTWAREClassesCLSID{00020D75-0000-0000-C000-000000000046}]
[HKEY_LOCAL_MACHINESOFTWAREClassesCLSID{00020D75-0000-0000-C000-000000000046}ShellFolder]
“Attributes”=dword:00000072
[HKEY_LOCAL_MACHINESOFTWAREClassesCLSID{00020D75-0000-0000-C000-000000000046}]
@=”Microsoft Office Outlook”
“InfoTip”=”Displays your e-mail, calendar, contacts, and other important personal information.”
[HKEY_LOCAL_MACHINESOFTWAREClassesCLSID{00020D75-0000-0000-C000-000000000046}DefaultIcon]
@=”C:\PROGRA~2\MICROS~1\Office12\OUTLOOK.EXE,7″
[HKEY_LOCAL_MACHINESOFTWAREClassesCLSID{00020D75-0000-0000-C000-000000000046}InprocServer32]
@=”C:\PROGRA~2\MICROS~1\Office12\MLSHEXT.DLL”
“ThreadingModel”=”Apartment”
[HKEY_LOCAL_MACHINESOFTWAREClassesCLSID{00020D75-0000-0000-C000-000000000046}Shell]
[HKEY_LOCAL_MACHINESOFTWAREClassesCLSID{00020D75-0000-0000-C000-000000000046}ShellOpen]
[HKEY_LOCAL_MACHINESOFTWAREClassesCLSID{00020D75-0000-0000-C000-000000000046}ShellOpenCommand]
@=””C:\PROGRA~2\MICROS~1\Office12\OUTLOOK.EXE””
[HKEY_LOCAL_MACHINESOFTWAREClassesCLSID{00020D75-0000-0000-C000-000000000046}ShellPropertiescommand]
@=”rundll32.exe shell32.dll,Control_RunDLL “C:\PROGRA~2\MICROS~1\Office12\MLCFG32.CPL””
[HKEY_LOCAL_MACHINESOFTWAREClassesCLSID{00020D75-0000-0000-C000-000000000046}shellex]
[HKEY_LOCAL_MACHINESOFTWAREClassesCLSID{00020D75-0000-0000-C000-000000000046}shellexPropertySheetHandlers]
[HKEY_LOCAL_MACHINESOFTWAREClassesCLSID{00020D75-0000-0000-C000-000000000046}shellexPropertySheetHandlers{00020D75-0000-0000-C000-000000000046}]
@=””
[HKEY_LOCAL_MACHINESOFTWAREClassesCLSID{00020D75-0000-0000-C000-000000000046}ShellFolder]
“Attributes”=hex:72,00,00,00
[HKEY_LOCAL_MACHINESOFTWAREMicrosoftWindowsCurrentVersionExplorerDesktopNameSpace{00020D75-0000-0000-C000-000000000046}]
You can save above bunch of keys as reg file and import into the target machine. Obvious thing is that you should take backup of your registry before you import.
Hope this tip helps you!!
[Source]
{ }
by TechiBee
on July 29, 2010
[PLEASE USE https://techibee.com/powershell/check-disk-space-of-remote-machine-using-powershell/430 to check free disk space of remote computer. I made it more advanced there.]
Description:
This function takes the computer name as argument and displays the disk free/total size information. One advantage with this function is, no need to search for admin windows when you want to run it. It prompts for admin credentials and connects to remote computer using them.
Usage:
PS C:temp> Check-Diskspace -computer remotepc
cmdlet Get-Credential at command pipeline position 1
Supply values for the following parameters:
Credential
Drive Name : C:
Total Space : 232.83 GB
Free Space : 0.94 GB
PS C:temp>
Code:
function Check-Diskspace {
param (
[parameter(Mandatory = $true)]
[string]$computer
)
$cred = Get-Credential
$drives = Get-WMIObject -Class Win32_LogicalDisk -ComputerName $computer -Credential $cred | where { $_.DriveType -eq 3 }
foreach ($drive in $drives) {
write-host "Drive Name : " $drive.DeviceID
write-host "Total Space : "($drive.size/1GB).ToString("0.00") "GB"
write-host "Free Space : " ($drive.FreeSpace/1GB).ToString("0.00") "GB"
write-host " "
}
$cred = $null
}
{ }
by TechiBee
on July 28, 2010
Simple Way to get local computer uptime….
PS C:> (gwmi win32_operatingSystem).lastbootuptime
20100728180723.375199+330
PS C:>
Now, let’s query the remote computers uptime.
PS C:> (gwmi win32_operatingSystem -computer remotePC).lastbootuptime
20100728180723.375199+330
PS C:>
The above are oneliners, if you want to convert this into full fledged function, use the below code. This gives the uptime of computer you have parsed.
C:>Get-PCuptime -computer remotePC
System(remotePC) is Uptime since : 14 days 20 hours 54 minutes 20 seconds
C:>
Code for function:
function Get-PCUptime {
[cmdletbinding()]
param($computer)
$lastboottime = (Get-WmiObject -Class Win32_OperatingSystem -computername $computer).LastBootUpTime
$sysuptime = (Get-Date) – [System.Management.ManagementDateTimeconverter]::ToDateTime($lastboottime)
Write-Host“System($computer) is Uptime since : ” $sysuptime.days “days” $sysuptime.hours `
“hours” $sysuptime.minutes “minutes” $sysuptime.seconds “seconds”
}
{ }
by TechiBee
on July 28, 2010
Changing the password is one of the regular activities for a typical System Administrator. It is not an easy task unless you have required tools in your tool kit. If you have changed one of your domain admin password, you need to find the places(be it services or scheduled tasks) where you have saved it and update password there. If you have 1000 servers, then you will endup updating/scanning remote server’s services/scheduled tasks one after another unless you have some home grown scripts to serve this purpose.
Well, I have some good news if you are still spending long hours to change the passwords. Today I came across a new utility called SSTUM (Service and Scheduled Task User Manager) which helps you in changing the password in remote services or scheduled Tasks with less efforts.
A few things I notices about this tool are…
1) Easy to use and saves lot of time
2) Input can be a computer list or you can make selection from active directory
3) Has an option to restart service after changing the password — pretty useful
4) Support exporting of results to some text file
5) Supports changing the username as well.
More details about this tool and download location are available at http://martin77s.wordpress.com/2010/07/19/service-and-scheduled-task-user-manager/
Hope this definitely helps.
{ }
by TechiBee
on July 25, 2010
For one of our client, we have received an alert stating that “The Microsoft Exchange Information Store service terminated with service-specific error 0 (0x0)”.
The error seems to simple and everyone would just suggest to start the service back. But the root cause was different.
One of our colleague started working on the alert and here is the chronology of steps followed in resolving the issue. found that the Information Store service was in stopped state and started the service. Started verifying queues , encoutered error stating that “Default SMTP Virtual Server is unavailable”. Verified and found that SMTP Service was in started mode.
Escalated call to me and I’ve started working on the issue.
Upon further analysis, found that the SMTP Virtual Instance was stopped in ESM (Exchange System Manager). Tried to start the instance, encountered error stating that “Queue Directory is corrupted , hence the instance could not be started”.
Error logged in Eventlog & Error pop-up when accessed Queue Directory from explorer.
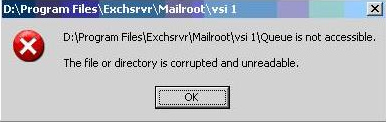
Executed following Steps to resolve issue:
1. Uninstalled existing Antivirus (AVG)
2. Executed chkdsk on volume in which the exchange database is stored. Found disk errors.
3. Executed chkdsk /f on the volume and restarted the server
4. Created new Queue Directory and pointed the path from ESM to the new folder.
5. Started SMTP Virtual Instance & Information Store Services.
Mails started flowing fine.
{ }
by TechiBee
on July 23, 2010
Outlook was unable to recover some or all of the items in this folder. Make sure you have the required permissions to recover items in this folder and try again. If the problem persists, contact your administrator
I came across this error while I(or my users) trying to restore bulk emails in outlook using “Recover Deleted Items” option. Single or little more emails restoration works fine but this issue is coming only when I attempt bulk restoration.
When this happened for the user, I am clue less what was happening. I went to event viewer on the server where user mailbox is hosted and observed below error when the user is attempting the restoration.
Event Type: Error
Event Source: MSExchangeIS
Event Category: General
Event ID: 9646
Date: 7/19/2010
Time: 3:45:47 PM
User: N/A
Computer: MYEXCHANGE
Description:
Mapi session “/o=ORG/ou=SITE/cn=Recipients/cn=myuser” exceeded the maximum of 500 objects of type “objtFolder”.
After a little bit of googling I found an article (http://support.microsoft.com/kb/830829/en-us) which is talking about similar issue and increasing the limits on Object types that you see in event viewer should fix the issue. I tried increasing the ObjFolder limit to 1000(note: 500 is the default; if you don’t find this key, it is safe to create one as per KB) on my exchange server but still it didn’t work and was saying exceeded the limit of 1000 this time. At this point I was not sure what was the optimum value to which I should increase this limit to make the restoration happen. As I didn’t find answer to this question in google, I polled one of friend who is with MS and knows more about exchange. He said there are no limits defined as such and it’s a trial and error method we should try. I bumped it to 10,000 this time and restoration worked. Along with this informaiton, my friend passed me a note as well. The more limit you put the more the load on your exchange server. So, be cautious while increasing the limits and I prefer to do it during off hours so that load will be less on the server and your users will be away anyways and that also gives you ample time to troubleshoot any unforeseen issues.
Additional note: While trying this you might see events about exceeding the limits of other object types described in aforementioned KB. You should create necessary registry keys for them as well to make your restoration suceessfull. Don’t forget to revert your settings once you are done with restoration; otherwise it will impact your server performance. Generally this will come into effect after store restart. Otherwise you can wait for 15-30 mins to make store pick these changes without restarting the IS.
Hope this information helps you.
{ }