In this post, I will show you how to convert a number in string format to integer. Before we jump further and see how to do it, I want to begin with help you understand why we need to do it. If a number is stored as a string, you can cannot perform addition or any other numeric operations on that variable until you convert it to integer or any other numeric types.
Let us see an example of what I am mean. As you can see from the below screenshot, a number(100) is stored in $string variable. When I perform an addition operation on it expecting the value to increase to 110, but it appended 10 to 100 in string fashion and returning the value of 10010. So, it is necessary to convert to a numeric value before we perform addition.
$string="100" $string + 10

Converting a string to number is very easy. For this purpose I am using Parse() method in System.Int32 class.
$string = "100" $numval = [int]::Parse($string) $numval $numval.gettype() $numval + 10
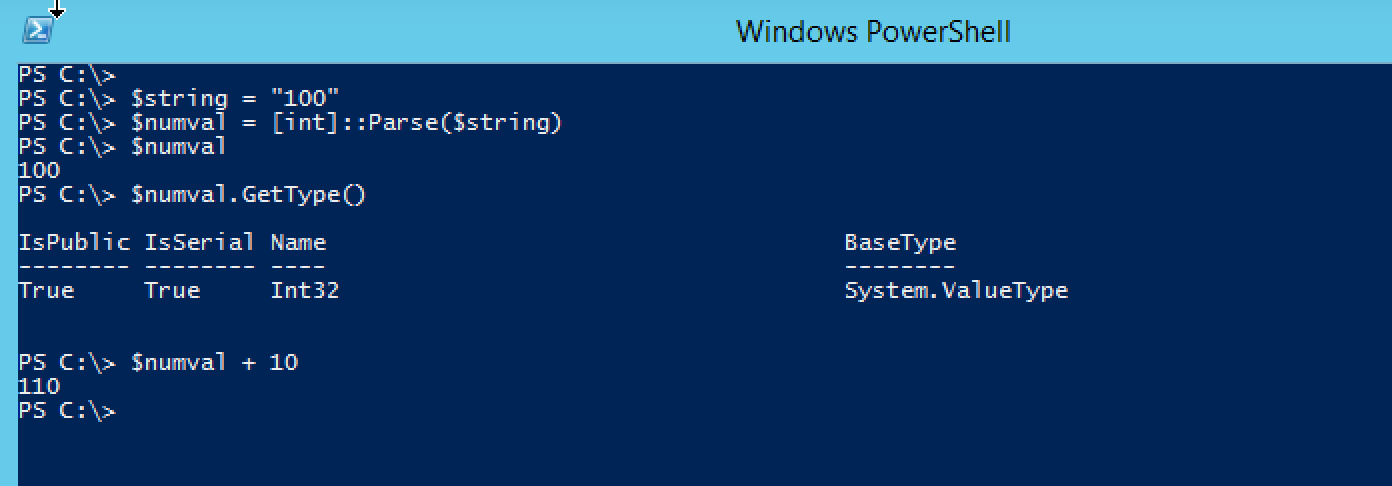
As you can see from the output, the $numval variable is a int32 type and now the addition operation on the value is working as expected.
Hope this helps.
Comments on this entry are closed.
I use the `-as` operator to cast one type into another. Example,
> $letter = “5”
> $letter | Get-Member
… returns
TypeName: System.String [etc., etc.]
> $number = $letter -as [int]
> $number | Get-Member
… returns
> TypeName: System.Int32 [etc., etc.]
HTH,
/Acey