In previous post we have seen how to get SID of current logged on user account using PowerShell. In this post, I will show you how to find if the current logged user account is a local account or a Domain level account.
Related Posts
This previous post we have used UserPrincipal class to get the current logged details. In this post we will use the object returned from this and find out if the user account is local user account or Domain User.
First let us get the details of UserPrincipal into a variable. Don’t forget to load the assembly before accessing AccountManagement namespace.
Add-Type -AssemblyName System.DirectoryServices.AccountManagement $UserPrincipal = [System.DirectoryServices.AccountManagement.UserPrincipal]::Current
One of the properties of the returned object is ContextType which contains the details we are looking for. If the value of this property is Domain then the user account is a domain account. The value will be Machine if the account is a local user account.
$UserPrincipal.ContextType
We can write a small wrapper around this and make a small function that can be reused in any script. This function returns either DomainUser or LocalUser string based on the account type.
Function Get-CurrentUserType { [CmdletBinding()] Param( ) #Import Assembly Add-Type -AssemblyName System.DirectoryServices.AccountManagement $UserPrincipal = [System.DirectoryServices.AccountManagement.UserPrincipal]::Current if($UserPrincipal.ContextType -eq "Machine") { return "LocalUser" } elseif($UserPrincipal.ContextType -eq "Domain") { return "DomainUser" } }
Copy this function into PowerShell window and invoke the function to see the results.
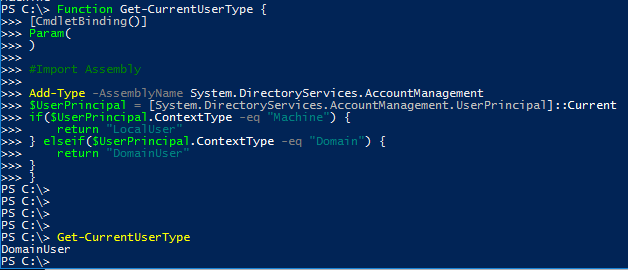
Do you have any questions about this approach? Write it in comments section.