In this short post, let us see how to query list of listening ports in local computer using PowerShell without using netstat.exe.
We know that below simple command will help in querying the list of listening ports, but capturing the output and filtering the output for specific IPs/ports is not straightforward with this approach.
So let us see how to achieve this without using netstat.exe and by relying on dotnet methodologies. The System.Net.NetworkInformation.IPGlobalProperties class contains the information about these listening ports as well. In my previous post, I talked about how to get list of active ports using same dotnet class.
READ : Query list of active TCP connections in Windows using PowerShell
The GetActiveTcpListeners() method will return list of listening connections, local IP addresses, and the port they are listening on. The below code will return this information in object format so that information can be easily filtered to fetch the desired output.
CODE
Function Get-ListeningTCPConnections { [cmdletbinding()] param( ) try { $TCPProperties = [System.Net.NetworkInformation.IPGlobalProperties]::GetIPGlobalProperties() $Connections = $TCPProperties.GetActiveTcpListeners() foreach($Connection in $Connections) { if($Connection.address.AddressFamily -eq "InterNetwork" ) { $IPType = "IPv4" } else { $IPType = "IPv6" } $OutputObj = New-Object -TypeName PSobject $OutputObj | Add-Member -MemberType NoteProperty -Name "LocalAddress" -Value $connection.Address $OutputObj | Add-Member -MemberType NoteProperty -Name "ListeningPort" -Value $Connection.Port $OutputObj | Add-Member -MemberType NoteProperty -Name "IPV4Or6" -Value $IPType $OutputObj } } catch { Write-Error "Failed to get listening connections. $_" } }
OUTPUT
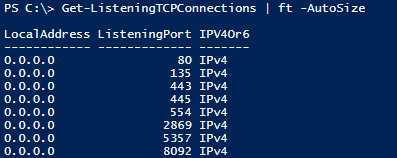
Hope this helps…
Comments on this entry are closed.
This helps a lot. Thanks for sharing this.
Glad to know that.
Hello,
many thank for your help!
But how can I get to open port 23? I dont see him.
Thank Sir
@r
Hey,
I’m really not sure why they don’t have this functionality built in. Super useful.
Cheers,
Justin!
Hi Justin, You can get similar output using Get-NetConnection cmdlet.
Get-NetTCPConnection | ? {$_.State -eq “Listen” }