You may experience slow internet connection on your Windows 10 PC when you have more than one active network connection. This article helps you to resolve such issues and make the internet browsing experience faster.
You might wondering why a computer need multiple network connection, but yes, it is a possible scenario. Some people use Wi-Fi connectivity for browsing and LAN connection for connecting to work place network etc. So, it is quite a possible scenario.
The issue will surface when one of the connections has internet access and other connection doesn’t have. You will start noticing internet connection taking more time and your browser spends time at Resolving host state(you can notice this chrome) during this process. If you ask any Windows expert, they will say change the network order to ensure adapter with network connection is first in the list.
In older version of windows, there is a concept of network adapter order. That means OS will chose first one in the list to perform network related operations and if it fails goes to the next one. See below image for better understanding.
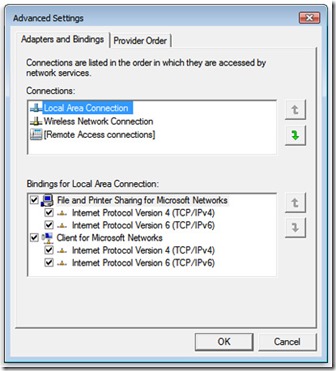
Image source: Microsoft Networking Blog
But this is improved in Windows 10 and now there no concept of ordering the network as OS is capable of taking the intelligent decisions based on a few factors. However when it comes to DNS name resolution, the components still looks for ordering (source: https://blogs.technet.microsoft.com/networking/2015/08/14/adjusting-the-network-protocol-bindings-in-windows-10/) and they use InterfaceMetric property of the adapter to make the choice. The lower this number, the higher the preference for dns name resolution.
So, this is problem we are hit with. Generally Wi-Fi networks InterfaceMetric value is more than LAN adapter value. So, the name resolution process will try to use the DNS servers configured in LAN adapter first to resolve the internet names like google.com etc. When it fails, then it will goto the next adapter i. e Wi-Fi adapter. The problem is worse when you have more than 2 adapters (possible when you install Hyper-V role on Windows 10).
I tried following the suggestion at Microsoft blog(https://blogs.technet.microsoft.com/networking/2015/08/14/adjusting-the-network-protocol-bindings-in-windows-10/) but it didn’t work for me. I believe the logic in that blog to determine the existing order is incorrect. So, I did a bit of experimenting and came up with below approach which worked great for me. In my case, I have more than 2 adapters as I am running Hyper-V role.
First we need to check what is the existing metric values for the adapters. We can do it by running below command from a PowerShell window with Administrator rights. Make a note of the current values so that you can revert to original settings if the fix is not working for you.
Get-NetIPInterface -AddressFamily IPv4 | select ifIndex,ifAlias,interfaceMetric
As you can see, my Wi-fi adapter metric is 50 and there are other adapters that value less than 50. That means Operating System will first try to resolve the name using the DNS servers configured on those adapters and it comes to Wi-Fi if it gets no response. Since they are private networks, resolving internet names will not work through them. Now we need to change the metric to a value lower than these adapters. So, I decided to set the metric value of Wi-Fi adapter to 10 so that it becomes less than other adapters. I have used below command to do this.
Set-NetIPInterface -InterfaceAlias "Wi-fi" -InterfaceMetric 10
Once this is executed without issues, you can restart the box and you will notice internet experience is back to normal. If not, revert back to old value to running the same command with original InterfaceMetric Value.
Please note that this is personal experience and may or may not work in your case. So, understand the scenario of your problem and decide if this fix will help you. Please write in comments section if this fix helped you or not. If you know any other fix, please share it.