This post will provide a script that you can use to perform password reset for multiple Active Directory user accounts at one go. This you can perform irrespective of the user account’s location in Active Directory or domain they are in. This simple script also generates a CSV file with the results of password reset.
This script takes 2 arguments. First one is list(array) of user accounts and second argument is domain name to which these user accounts belongs to. The first argument is mandatory, and you should provide at least one user name. Otherwise script will not proceed. Second argument, domainname, is optional. By default, it assumes that all the user accounts you provided are present in the same domain as the account that is running the script.
Since this script doesn’t need much of explanation, we can jump straight into how to execute this script. Btw, this script relies on ActiveDirectory PowerShell module and uses Set-ADAccountPassword cmdlet to reset the password. If you don’t have this module, the script will fail to reset the password.
Script will prompt you to enter the password and confirm it again. It performs the comparison and ensures that you have entered it correctly both the times. It also checks that password is not less than 8 characters long. In both cases, it will exit with appropriate error message. So, make sure you are meeting required password complexity requirements. The checks here are simple ones and you can add more checks if you want.
Code:
Copy the below code into a file called Reset-UserPassword.ps1 and save it.
[CmdletBinding()] param( [Parameter(Mandatory=$True)] [string[]]$UserName, [string]$DomainName = $env:USERDOMAIN ) #Logic to read password $password1 = Read-Host "Enter the password you want to set" -AsSecureString $password2 = Read-Host "Confirm password" -AsSecureString $pwd1_plain = (New-Object PSCredential "user",$password1).GetNetworkCredential().Password $pwd2_plain = (New-Object PSCredential "user",$password2).GetNetworkCredential().Password if($pwd1_plain -ne $pwd2_plain) { Write-Host "Either passwords didn't match" -ForegroundColor Yellow return } if($pwd1_plain.Length -lt 8) { Write-Host "Password length is less than 8 characters. Make sure you are meeting complexity requirements" -ForegroundColor Yellow return } $DEFAULT_PASSWORD = $password1 $OutCSV = @() foreach($user in $UserName) { $OutputObj = New-Object -TypeName PSobject $OutputObj | Add-Member -MemberType NoteProperty -Name DateTime -Value $(Get-Date) $OutputObj | Add-Member -MemberType NoteProperty -Name DomainName -Value $DomainName $OutputObj | Add-Member -MemberType NoteProperty -Name UserName -Value $User $OutputObj | Add-Member -MemberType NoteProperty -Name ResetBy -Value $env:USERNAME $OutputObj | Add-Member -MemberType NoteProperty -Name ResetStatus -Value $null Write-Verbose "$User : Working on retting the password" Write-Verbose "$User : Searching for the user in Active Directory" try { Set-ADAccountPassword -identity $User -Server $DomainName -Reset -NewPassword $DEFAULT_PASSWORD -EA Stop Write-Verbose "$User : Successfully Changed the password" Write-Host "$User : Password reset successfully" -ForegroundColor Green $OutputObj.ResetStatus = "SUCCESS" } catch { Write-Verbose "$user : Error occurred while resetting the password. $_" Write-Host "$User : Password reset FAILED" -ForegroundColor Yellow $OutputObj.ResetStatus = "FAILED : $_" } $OutCSV += $OutputObj } $outputfile = "c:\temp\PasswordResetStatus.csv" Write-Host "Output is stored at $outputfile" $OutCSV | export-csv -Path $outputfile -NoTypeInformation -Force
Usage:
Now us see the ways you can use this script. Since all the user accounts we are located in techibee.local domain, I am passing it to the -DomainName parameter.
Reset a single user password
.\Reset-UserPassword.ps1 -UserName testuser1 -DomainName techibee.local
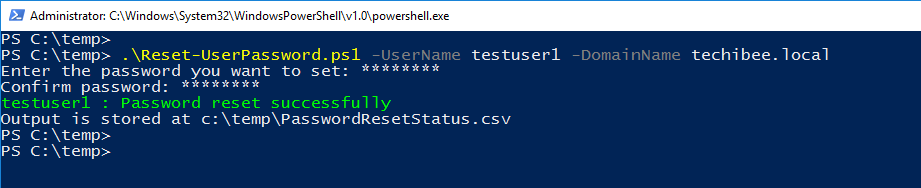
Reset multiple user’s passwords
.\Reset-UserPassword.ps1 -UserName testuser1,testuser2,testuser3 -DomainName techibee.local
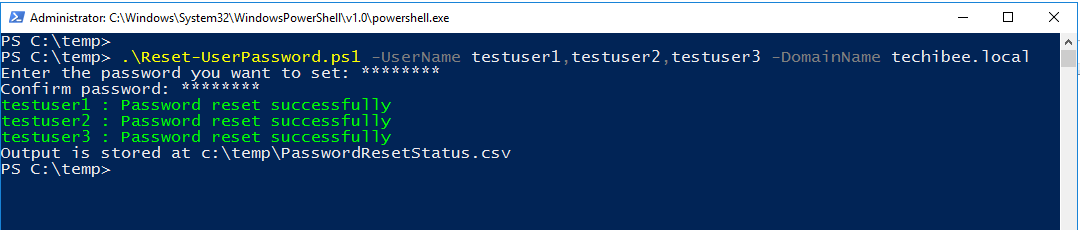
Reset multiple user’s passwords by reading user lists from text file.
$users = Get-Content C:\temp\users.txt .\Reset-UserPassword.ps1 -UserName $users -DomainName techibee.local
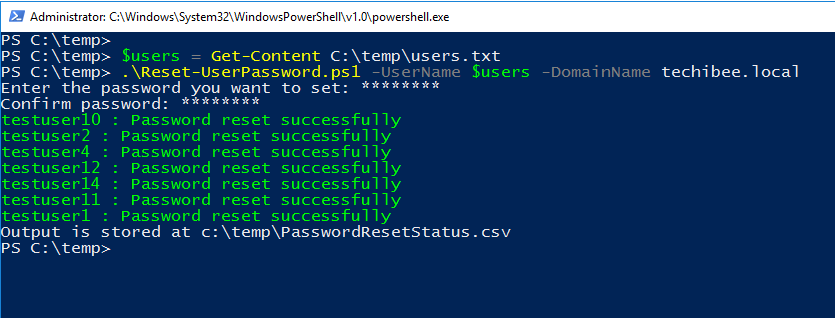
The output CSV generated by the script looks like below.
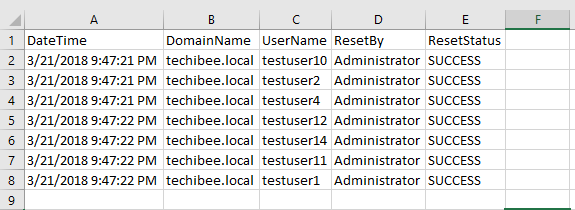
Hope this helps. Let us know if you have a requirement that you want to embed in the script. We will try accommodating it on best efforts basis. Please write in comments section.
Comments on this entry are closed.
hello, nice job
how to use searchabase on this script for reset password users on specific ou ?
thank for answer.
How to reset paswords for the ones whole status is False or else for the users for whom the script failed to reset password