In this article, we find out how to use PowerShell to read a list of IP addresses from a text file and resolve them to hostnames and then export to a CSV file for further reviewing.
A lot of systems like Windows Event logs, Firewall logs or IIS logs etc will store the connection information with IP addresses. Barely looking at the IP address may not give a good idea of what systems are connecting and resolving them to hostnames is much easier to process the data. The script that we are going to discuss will read a list of IP addresses from a text file, resolves them to hostnames, and finally exports them to CSV file.
We are going to use [System.Net.DNS] DotNet class for this purpose. The GetHostbyAddress() method available in this class will help us in resolving names to IP addresses.
Code
[CmdletBinding()]
param(
[Parameter(Mandatory=$True)]
[string[]]$IPAddress,
[switch]$ExportToCSV
)
$outarr = @()
foreach($IP in $IPAddress) {
$result = $null
try {
$Name = ([System.Net.DNS]::GetHostByAddress($IP)).HostName
$result = "Success"
} catch {
$result = "Failed"
$Name="NA"
}
$outarr += New-Object -TypeName PSObject -Property @{
IPAddress = $IP
Name = $Name
Status = $result
} | Select-Object IPAddress, Name, Status
}
if($ExportToCSV) {
$outarr | export-csv -Path "c:\temp\iptoname.csv" -NoTypeInformation
} else {
$outarr
}
Some of the readers of my blog might ask why to use GetHostbyAddress method when Resolve-DNSName can do the task. It is simple; the .Net method works from most of the windows computers in use today, including Windows XP. Using Resolve-DNSName requires you to run from Windows8/Windows Server 2012 or above computers. However, use Resolve-DNSName where you can, because it is simply the latest and lets you query other types of records too. The .Net method lets you query A, AAA and PTR records only.
Usage
You can use this script by passing list of IP addresses to -IPAddress parameter as shown below. The Name column indicates the name the ip resolved to, and the Status column contains whether name resolution is successful or not.
.\Resolve-IpToName.ps1 -IPAddress 69.163.176.252,98.137.246.8
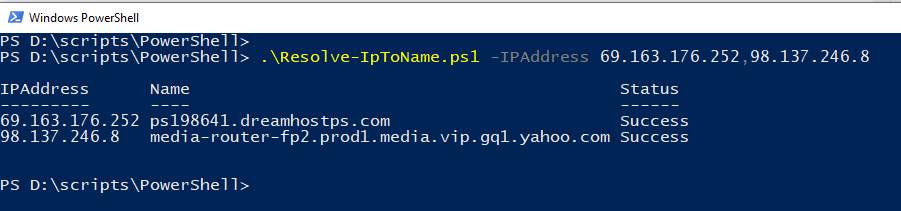
You can input the list of IPAddress using text file as well.
.\Resolve-IpToName.ps1 -IPAddress (get-content c:\temp\ips.txt)
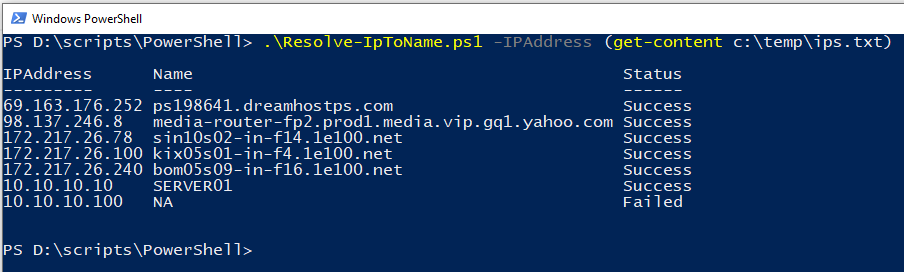
By default, the script shows the output on the screen. If you want to export them to CSV, use -ExportToCSV switch.
.\Resolve-IpToName.ps1 -IPAddress (get-content c:\temp\ips.txt) -ExportToCsv
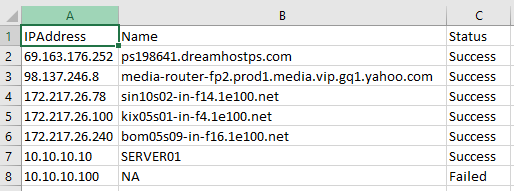
Above CSV file gets generated when you use -ExportToCSV switch.
Hope you find this article useful. Please write in the comment section if you are looking for additional functionality. We will try to make it on the best effort basis.
Comments on this entry are closed.
Nice work !
I just have a comment. I’m always embarrassed when in a script I see a variable or apath hard-coded (I.E. iptoname.csv)
You should change the variable type for $ExportToCsv front [Switch] to [String] and so we could setup a path as a parameter
Regards
Olivier
Thank you, Olivier, for the feedback. I understand how bad it looks when some of the inputs are hardcoded. I will update that. Btw, if you want to export to different CSV, you can always use Export-CSV cmdlet.
Hi,
Thanks for the script. I beginner in Powershell and got error code :
Resolve-IPToName.ps1 : Cannot bind argument to parameter ‘IPAddress’ because it is an empty string.
At line:1 char:35
Can you help me. I think my ips.txt is not good.
Regards.
Michel