In this post, we are going to learn how to test if a given variable has numbers in it using PowerShell. There are two scenarios here to cover. First one is checking if the whole value is number and second scenario is checking if part of the value is number and rest are characters or any other symbols.
Let us talk about our first case, checking if given string variable contains a number. Look at the below example. The variable $myvar has a value “123”. Though it is a numeric number, PowerShell sees it as a String.
$myvar = "123" $myvar -is [int] $myvar.gettype()

So how do we determine if the value contains the number? Well, here regular expressions comes handy. Since the value is a string type we can use a regex to very if this string value is completely a number or not.
$myvar = "123" $myvar -match "^\d+$"
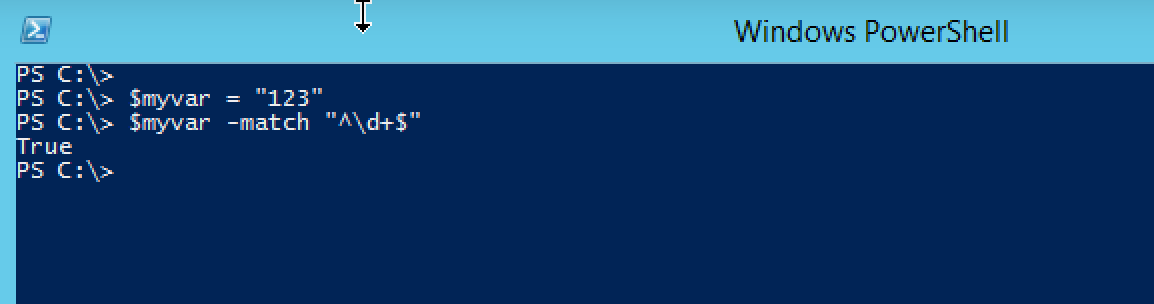
As you can see it returned true which means this string contains number only. The regex I used is a very simple one which checks if there are digits in the given string(\d+) and ^,$ at the beginning and ending represents that should start with a number and end with a number. This satisfies all the required rules to figure out if a string value is number or not.
Now, how do we deal with a case where the string is mix of numbers and characters. There is a solution for it. Take the below example.
$myvar = "edf123xyz" $myvar -match ".*\d+.*"
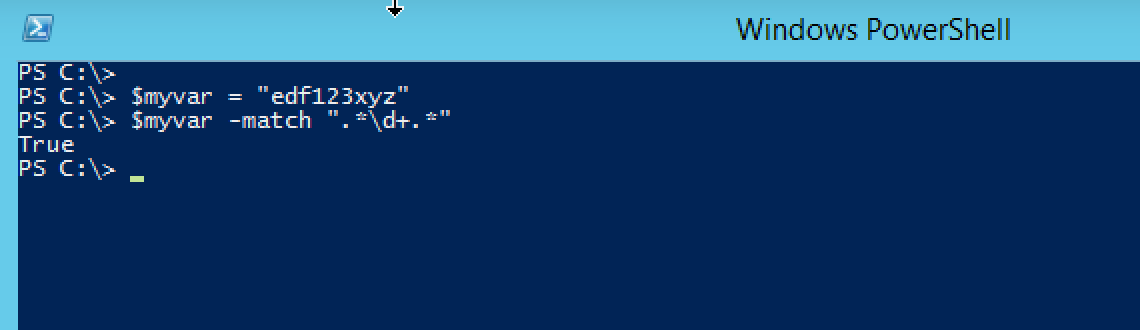
Here I am using another regex but in a different way. I am checking if the given string has numbers anywhere in the string. This is sufficient enough to very if given string value has numbers in it.
Hope this helps.
Comments on this entry are closed.
This helped a lot, thank you!
thanks, your hint solved my question – how to check an array for only numbers:
$numbersInArray = $(@(“1″,”2″,”3″,”not a number”) -match “\d+$” | measure)
if ($numbersInArray.Count -eq @(“1″,”2″,”3″,”not a number”).Count) { Write-Host “only numbers in array” }
# nope, but:
$numbersInArray = $(@(“1″,”2″,”3”) -match “\d+$” | measure)
if ($numbersInArray.Count -eq @(“1″,”2″,”3”).Count) { Write-Host “only numbers in array” }